Data types check in JavaScript- JavaScript Data Types
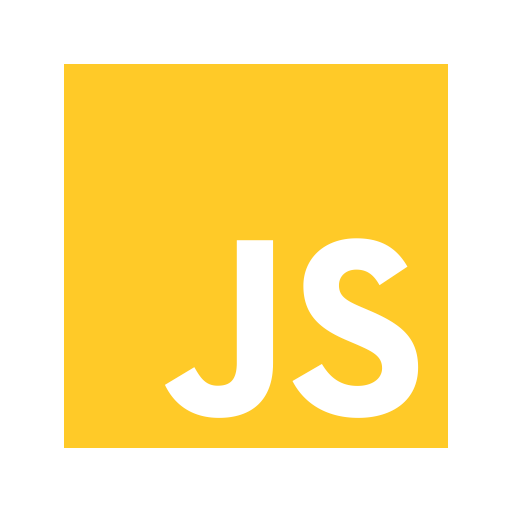
JavaScript has an operator called typeof to check what data type something has. It has very easy syntax to use. typeof operator returns the string of the data type of the value. i.e “string” is returned for data type String and “object” for Object. typeof operator isn’t perfect. But we can use it for primitive data types.
Data Types
String
A string can be a simple “string” or the instance of the String object. In case of string called with new String operator, typeof operator will return “object”. So, to get the actual data type, “instanceof” can be used.
function isString (value) { return typeof value === 'string' || value instanceof String; }
Number
Other than actual number, typeof operator will return “number” for other data too, like NaN and Infinity. For such conditions, we use isFinite function with typeof operator.
function isNumber (value) { return typeof value === 'number' && isFinite(value); }
Object
To check something is an object, we can compare its constructor to Object along with typeof operator. instanceof operator can also be used for objects created from classes.
function isObject (value) {
return value && typeof value === 'object' && value.constructor === Object;
}
Array
Arrays are not true arrays in Javascript, like in other programming languages. They’re objects so typeof will return “object” for arrays. To check if something’s really an array, we can compare their constructors with Array.
function isArray (value) {
return value && typeof value === 'object' && value.constructor === Array;
}
In ES5, it has a method to check array
Array.isArray(value);
Boolean
For boolean type check, typeof operator will do.
function isBoolean (value) {
return typeof value === 'boolean';
}
Date
Date object it can be checked with instanceof.
function isDate (value) {
return value instanceof Date;
}
Function
We can check functions as function with typeof.
function isFunction (value) {
return typeof value === 'function';
}
Null & undefined
Null and undefined values can be checked as follows:
// Returns if a value is null function isNull (value) { return value === null; }
// Returns if a value is undefined function isUndefined (value) { return typeof value === 'undefined'; }
Error
Errors in JavaScript are like “exceptions” in other programming languages. We can check with instanceof operator to check for Error type.
function isError (value) { return value instanceof Error && typeof value.message !=='undefined'; }
RegExp
RegExp’s are also objects, so we have to compare the constructor to be RegExp for RegExp.
function isRegExp (value) {
return value && typeof value === 'object' && value.constructor === RegExp;
}
More in-depth documentation of JavaScript data types can be found at https://developer.mozilla.org/en-US/docs/Web/JavaScript/Data_structures.